The QSlotObject is a wrapper around the slot that will help calling it. It also knows the type of the signal arguments so it can do the proper type conversion. We use ListLeft to only pass the same number as argument as the slot, which allows connecting a signal with many arguments to a slot with less arguments. The connection mechanism uses a vector indexed by signals. But all the slots waste space in the vector and there are usually more slots than signals in an object. So from Qt 4.6, a new internal signal index which only includes the signal index is used. While developing with Qt, you only need to know about the absolute method index.
Qt's meta-object system provides a mechanism to automatically connect signals and slots between QObject subclasses and their children. As long as objects are defined with suitable object names, and slots follow a simple naming convention, this connection can be performed at run-time by the QMetaObject::connectSlotsByName.
This blog is part of a series of blogs explaining the internals of signals and slots.
In this article, we will explore the mechanisms powering the Qt queued connections.
Summary from Part 1
In the first part, we saw that signalsare just simple functions, whose body is generated by moc. They are just calling QMetaObject::activate
, with an array of pointers to arguments on the stack.Here is the code of a signal, as generated by moc: (from part 1)
QMetaObject::activate
will then look in internal data structures to find out what are the slots connected to that signal.As seen in part 1, for each slot, the following code will be executed:
So in this blog post we will see what exactly happens in queued_activate
and other parts that were skipped for the BlockingQueuedConnection
Qt Event Loop
A QueuedConnection
will post an event to the event loop to eventually be handled.
When posting an event (in QCoreApplication::postEvent
),the event will be pushed in a per-thread queue(QThreadData::postEventList
).The event queued is protected by a mutex, so there is no race conditions when threadspush events to another thread's event queue.
Once the event has been added to the queue, and if the receiver is living in another thread,we notify the event dispatcher of that thread by calling QAbstractEventDispatcher::wakeUp
.This will wake up the dispatcher if it was sleeping while waiting for more events.If the receiver is in the same thread, the event will be processed later, as the event loop iterates.
The event will be deleted right after being processed in the thread that processes it.

An event posted using a QueuedConnection is a QMetaCallEvent
. When processed, that event will call the slot the same way we call them for direct connections.All the information (slot to call, parameter values, ..) are stored inside the event.
Copying the parameters
The argv
coming from the signal is an array of pointers to the arguments. The problem is that these pointers point to the stack of the signal where the arguments are. Once the signal returns, they will not be valid anymore. So we'll have to copy the parameter values of the function on the heap. In order to do that, we just ask QMetaType. We have seen in the QMetaType article that QMetaType::create
has the ability to copy any type knowing it's QMetaType ID and a pointer to the type.
To know the QMetaType ID of a particular parameter, we will look in the QMetaObject, which contains the name of all the types. We will then be able to look up the particular type in the QMetaType database.
queued_activate
We can now put it all together and read through the code ofqueued_activate, which is called by QMetaObject::activate
to prepare a Qt::QueuedConnection
slot call.The code showed here has been slightly simplified and commented:
Upon reception of this event, QObject::event
will set the sender and call QMetaCallEvent::placeMetaCall
. That later function will dispatch just the same way asQMetaObject::activate
would do it for direct connections, as seen in Part 1
BlockingQueuedConnection
BlockingQueuedConnection
is a mix between DirectConnection
and QueuedConnection
. Like with aDirectConnection
, the arguments can stay on the stack since the stack is on the thread thatis blocked. No need to copy the arguments.Like with a QueuedConnection
, an event is posted to the other thread's event loop. The event also containsa pointer to a QSemaphore
. The thread that delivers the event will release thesemaphore right after the slot has been called. Meanwhile, the thread that called the signal will acquirethe semaphore in order to wait until the event is processed.
It is the destructor of QMetaCallEvent which will release the semaphore. This is good becausethe event will be deleted right after it is delivered (i.e. the slot has been called) but also whenthe event is not delivered (e.g. because the receiving object was deleted).
A BlockingQueuedConnection
can be useful to do thread communication when you want to invoke afunction in another thread and wait for the answer before it is finished. However, it must be donewith care.
The dangers of BlockingQueuedConnection
You must be careful in order to avoid deadlocks.
Obviously, if you connect two objects using BlockingQueuedConnection
living on the same thread,you will deadlock immediately. You are sending an event to the sender's own thread and then are locking thethread waiting for the event to be processed. Since the thread is blocked, the event will never beprocessed and the thread will be blocked forever. Qt detects this at run time and prints a warning,but does not attempt to fix the problem for you.It has been suggested that Qt could then just do a normal DirectConnection
if both objects are inthe same thread. But we choose not to because BlockingQueuedConnection
is something that can only beused if you know what you are doing: You must know from which thread to what other thread theevent will be sent.

The real danger is that you must keep your design such that if in your application, you do aBlockingQueuedConnection
from thread A to thread B, thread B must never wait for thread A, or you willhave a deadlock again.
When emitting the signal or calling QMetaObject::invokeMethod()
, you must not have any mutex lockedthat thread B might also try locking.
A problem will typically appear when you need to terminate a thread using a BlockingQueuedConnection
, for example in thispseudo code:
You cannot just call wait here because the child thread might have already emitted, or is about to emitthe signal that will wait for the parent thread, which won't go back to its event loop. All the thread cleanup information transfer must only happen withevents posted between threads, without using wait()
. A better way to do it would be:

The downside is that MyOperation::cleanup()
is now called asynchronously, which may complicate the design.
Conclusion

This article should conclude the series. I hope these articles have demystified signals and slots,and that knowing a bit how this works under the hood will help you make better use of them in yourapplications.
Build complex application behaviours using signals and slots, and override widget event handling with custom events.
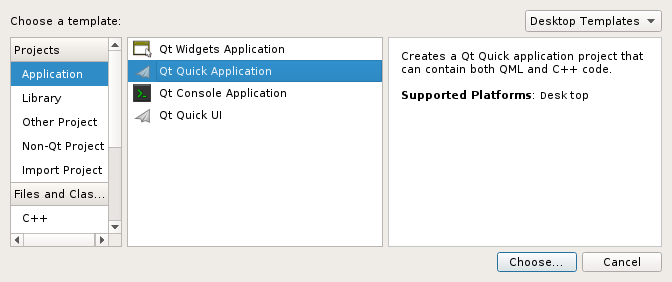
An event posted using a QueuedConnection is a QMetaCallEvent
. When processed, that event will call the slot the same way we call them for direct connections.All the information (slot to call, parameter values, ..) are stored inside the event.
Copying the parameters
The argv
coming from the signal is an array of pointers to the arguments. The problem is that these pointers point to the stack of the signal where the arguments are. Once the signal returns, they will not be valid anymore. So we'll have to copy the parameter values of the function on the heap. In order to do that, we just ask QMetaType. We have seen in the QMetaType article that QMetaType::create
has the ability to copy any type knowing it's QMetaType ID and a pointer to the type.
To know the QMetaType ID of a particular parameter, we will look in the QMetaObject, which contains the name of all the types. We will then be able to look up the particular type in the QMetaType database.
queued_activate
We can now put it all together and read through the code ofqueued_activate, which is called by QMetaObject::activate
to prepare a Qt::QueuedConnection
slot call.The code showed here has been slightly simplified and commented:
Upon reception of this event, QObject::event
will set the sender and call QMetaCallEvent::placeMetaCall
. That later function will dispatch just the same way asQMetaObject::activate
would do it for direct connections, as seen in Part 1
BlockingQueuedConnection
BlockingQueuedConnection
is a mix between DirectConnection
and QueuedConnection
. Like with aDirectConnection
, the arguments can stay on the stack since the stack is on the thread thatis blocked. No need to copy the arguments.Like with a QueuedConnection
, an event is posted to the other thread's event loop. The event also containsa pointer to a QSemaphore
. The thread that delivers the event will release thesemaphore right after the slot has been called. Meanwhile, the thread that called the signal will acquirethe semaphore in order to wait until the event is processed.
It is the destructor of QMetaCallEvent which will release the semaphore. This is good becausethe event will be deleted right after it is delivered (i.e. the slot has been called) but also whenthe event is not delivered (e.g. because the receiving object was deleted).
A BlockingQueuedConnection
can be useful to do thread communication when you want to invoke afunction in another thread and wait for the answer before it is finished. However, it must be donewith care.
The dangers of BlockingQueuedConnection
You must be careful in order to avoid deadlocks.
Obviously, if you connect two objects using BlockingQueuedConnection
living on the same thread,you will deadlock immediately. You are sending an event to the sender's own thread and then are locking thethread waiting for the event to be processed. Since the thread is blocked, the event will never beprocessed and the thread will be blocked forever. Qt detects this at run time and prints a warning,but does not attempt to fix the problem for you.It has been suggested that Qt could then just do a normal DirectConnection
if both objects are inthe same thread. But we choose not to because BlockingQueuedConnection
is something that can only beused if you know what you are doing: You must know from which thread to what other thread theevent will be sent.
The real danger is that you must keep your design such that if in your application, you do aBlockingQueuedConnection
from thread A to thread B, thread B must never wait for thread A, or you willhave a deadlock again.
When emitting the signal or calling QMetaObject::invokeMethod()
, you must not have any mutex lockedthat thread B might also try locking.
A problem will typically appear when you need to terminate a thread using a BlockingQueuedConnection
, for example in thispseudo code:
You cannot just call wait here because the child thread might have already emitted, or is about to emitthe signal that will wait for the parent thread, which won't go back to its event loop. All the thread cleanup information transfer must only happen withevents posted between threads, without using wait()
. A better way to do it would be:
The downside is that MyOperation::cleanup()
is now called asynchronously, which may complicate the design.
Conclusion
This article should conclude the series. I hope these articles have demystified signals and slots,and that knowing a bit how this works under the hood will help you make better use of them in yourapplications.
Build complex application behaviours using signals and slots, and override widget event handling with custom events.
As already described, every interaction the user has with a Qt application causes an Event. There are multiple types of event, each representing a difference type of interaction — e.g. mouse or keyboard events.
Events that occur are passed to the event-specific handler on the widget where the interaction occurred. For example, clicking on a widget will cause a QMouseEvent
to be sent to the .mousePressEvent
Superman slot machine apps to play. event handler on the widget. This handler can interrogate the event to find out information, such as what triggered the event and where specifically it occurred.
You can intercept events by subclassing and overriding the handler function on the class, as you would for any other function. You can choose to filter, modify, or ignore events, passing them through to the normal handler for the event by calling the parent class function with super()
.
However, imagine you want to catch an event on 20 different buttons. Subclassing like this now becomes an incredibly tedious way of catching, interpreting and handling these events.
Thankfully Qt offers a neater approach to receiving notification of things happening in your application: Signals.
Signals
Instead of intercepting raw events, signals allow you to 'listen' for notifications of specific occurrences within your application. While these can be similar to events — a click on a button — they can also be more nuanced — updated text in a box. Data can also be sent alongside a signal - so as well as being notified of the updated text you can also receive it.
The receivers of signals are called Slots in Qt terminology. A number of standard slots are provided on Qt classes to allow you to wire together different parts of your application. However, you can also use any Python function as a slot, and therefore receive the message yourself.
Load up a fresh copy of `MyApp_window.py` and save it under a new name for this section. The code is copied below if you don't have it yet.
Basic signals
Qt Connect Signal Parent Slot
First, let's look at the signals available for our QMainWindow
. You can find this information in the Qt documentation. Scroll down to the Signals section to see the signals implemented for this class.
Qt 5 Documentation — QMainWindow Signals
As you can see, alongside the two QMainWindow
signals, there are 4 signals inherited from QWidget
and 2 signals inherited from Object
. If you click through to the QWidget
signal documentation you can see a .windowTitleChanged
signal implemented here. Next we'll demonstrate that signal within our application.
Qt 5 Documentation — Widget Signals
The code below gives a few examples of using the windowTitleChanged
signal.
Try commenting out the different signals and seeing the effect on what the slot prints.
We start by creating a function that will behave as a ‘slot' for our signals.
Then we use .connect on the .windowTitleChanged
signal. We pass the function that we want to be called with the signal data. In this case the signal sends a string, containing the new window title.
If we run that, we see that we receive the notification that the window title has changed.
Events
Next, let's take a quick look at events. Thanks to signals, for most purposes you can happily avoid using events in Qt, but it's important to understand how they work for when they are necessary.
As an example, we're going to intercept the .contextMenuEvent
on QMainWindow
. This event is fired whenever a context menu is about to be shown, and is passed a single value event
of type QContextMenuEvent
.
To intercept the event, we simply override the object method with our new method of the same name. So in this case we can create a method on our MainWindow
subclass with the name contextMenuEvent
and it will receive all events of this type.
If you add the above method to your MainWindow
class and run your program you will discover that right-clicking in your window now displays the message in the print statement.
Sometimes you may wish to intercept an event, yet still trigger the default (parent) event handler. You can do this by calling the event handler on the parent class using super
as normal for Python class methods.
Qt Connect Parent Slot Machine
This allows you to propagate events up the object hierarchy, handling only those parts of an event handler that you wish.
However, in Qt there is another type of event hierarchy, constructed around the UI relationships. Widgets that are added to a layout, within another widget, may opt to pass their events to their UI parent. In complex widgets with multiple sub-elements this can allow for delegation of event handling to the containing widget for certain events.
However, if you have dealt with an event and do not want it to propagate in this way you can flag this by calling .accept()
on the event.
Alternatively, if you do want it to propagate calling .ignore()
will achieve this.
In this section we've covered signals, slots and events. We've demonstratedsome simple signals, including how to pass less and more data using lambdas.We've created custom signals, and shown how to intercept events, pass onevent handling and use .accept()
and .ignore()
to hide/show eventsto the UI-parent widget. In the next section we will go on to takea look at two common features of the GUI — toolbars and menus.